THIS TUTORIAL IS OUTDATED! Version 2.0 can be found hereBossesBosses are a very complicated subject. Previously, in stage design, it was more of a static process - you lay out everything as it's going to be in game, and then it's up to the player to navigate through your maze. Now, however, you'll be designing something that responds to the player, something that has phases and sequences, and it involves a strong familiarity with the object event/action editor.
In order to learn how a boss works, we'll make a generic one that does a little of everything; it will be up to you to extend these concepts and add your own ingenuity in order to make a boss that really stands out!
The first step in making your boss is going to be making a new object. Using the information you've learned so far, create a new sprite named sprMyBoss. We're going to use the image below for your boss. You can right-click it and save it to your computer, then load it into the sprite you just made using the "Create From Strip" option. Each half of this image is going to represent one frame of animation, and the individual images are 128x128. I promise I won't sue you for stealing my incredible artwork
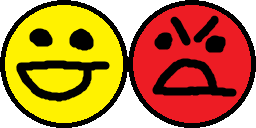
Now, make an object and name it objMyBoss, assigning it the sprite you just imported. You'll want to give this boss its own room, so go ahead and make one for it. It would also be a good idea to make a warp from the start of the game to this room, so you can test it easily! You'll want to move that warp later on, of course. Check the "Changing Rooms" section of the tutorial if you've forgotten how to do that. Once that's all set up, head back to the object editor for objMyBoss and let's get to work!
Giving your boss HPWhen it boils down to it, there are two kinds of bosses: ones you have to kill, and ones you have to survive. If you want an avoidance fight, you can skip this section. But for bosses that you want to shoot to kill, here's how you do it.
Add a
Create event, and add an
Execute Code action. Giving you boss HP is as simple as adding the following line of code:
hp = 20;
image_speed=0;
What this does is create a variable named
hp and set it to
20. There's no requirement to name your variable hp, we'll just use this because it's easy to remember (
warning: Do not name the variable "health" - this has a specific meaning in Game Maker and your boss will probably not work right if you do that. Go to Scripts->Show Built-In Variables in GM to see the names you cannot use for your own variables). You can also use any value other than 20; quit being so demanding and just go along with it for now!
The second line of code just makes your boss not change images from the happy to the angry face - we'll work on this later.
Alright, your boss has HP. But if you run your game, go into the room and shoot the boss, nothing will happen! Have I mislead you? No! There's nothing special about your bullets that makes them damage the boss; you'll have to set that up. In order to make bullets hurt your boss, go back into the object editor, and add a new event: Add Event->Collision->player_etc->bullet. This is a collision event, so it is fired every step that a bullet is touching your object, which is exactly what we want - when a bullet is touching the boss, damage it! So add the following code to the new bullet collision event:
hp -= 1;
sound_play(sndBossHit);
with (other) instance_destroy();
The -= operator is a common operator in programming; it's shorthand for
take the hp, subtract 1, and set hp to this new value. You could write
hp = hp - 1 and it would mean the exact same thing.
The second line plays a sound named
sndBossHit - this will play that iconic "thunk" sound when the bullet hits the boss. You can specify any other sound here; look through the
Sounds folder in GM to see what sounds are available, or to create your own epic sound of bullets ripping through flesh!
What about the third line? Let's break it down: the first half of the line states
with (other). The "with" keyword "shifts the scope" of following code. You place whatever object you want to run some code in the parentheses - "with (player)" would make the following code be ran by the
player object. We've specified
other, which is a GM Keyword meaning "the other instance involved in this event". A collision event involves two objects - the one whose event was triggered (the boss) and the one who triggered the event (the bullet). So we're shifting the scope to the bullet, and the following code will be ran by the bullet.
The code being run by the bullet is
instance_destroy() - a special GM function which does exactly what it says - removes the instance from the game. When the bullet collides with the boss, we don't want it to collide again on the next frame, so we have to destroy it. That's what this code does.
You could run your game again and go shoot your boss, and every time you shoot it, you should see your bullet disappear, and hear the thunk sound. But when you shoot it 20 times, he doesn't die! Again, you have to do this yourself; GM won't be helpful at all

To make the boss die after 20 shots, we'll have to augment our bullet collision event. Use the enhanced code below:
hp -= 1;
sound_play(sndBossHit);
if (hp == 0) {
sound_play(sndDeath);
instance_destroy();
}
with (other) instance_destroy();
Here, we've added a block of code that will check to see if the hp is zero now, and if so, kill the boss. Make sure to use the double-equal-sign to test if something is equal to something; if you use a single equals sign, it will just set the variable to zero!
The curly braces define a "code block" - since it comes right after the if statement, everything in the block will only be executed if the if statement turns out true - so when the hp is zero, it'll play the death sound and kill the boss.
NOW you can run your game kill your boss! But what good is a boss that just sits there and lets you kill him? No good at all!
Boss MovementThis section is a work in progress...Boss AttacksLet me start off by saying that things get real complicated here. We're going to be using a lot of coding and object editing, so be real comfortable with those before you go any further.
If you've made it this far, it means I haven't scared you off yet! very well, I shall confer to you the true meat of boss design - the attacks. In pretty much every boss fight - avoidance especially - patterns of apples and spikes fly all over the screen, while you stay alive. I'm going to show you a bunch of generic attack patterns, and will likely get a lot of flak for teaching everyone how to do the same boring stuff everyone else does... so
please, only use them as a starting point for your own amazing ideas!Before you make any of the below attacks, lets start with a little preparation. Open up the boss object from earlier and give him two events: Create and Alarm 0 (it's ok if he already has a create event). In the create event, add this code:
alarm[0]=1;
This code sets Alarm 0 to one tick (frame) after creation.
An alarm counts down by one every tick, and when it hits zero, it executes whatever you've placed inside its event. If you want to delay your boss's first attack, increase this value. Remember to base all your values off of
room_speed (the number of ticks per second), so for a delay of two seconds you would do:
alarm[0]=2*room_speed;
Also go ahead and make an object called objApple - this will be our projectile. Give it an apple sprite, and make its parent object "playerKiller" Finally, add an "outside room" event (under other) and add a Destroy Instance action (self). That's all, now let's move on to making the boss do stuff!
Your boss's projectile:
Targetted applesTargetted apples seem to be a staple of boss fights. These are really easy to make; in fact, there's just a couple of lines of code you need to add to Alarm 0:
apple=instance_create(x,y,objApple);
apple.speed=6;
apple.direction=point_direction(x,y,player.x,player.y);
alarm[0]=room_speed/2;
Line by line, this code creates an apple, sets its speed to 6, calculates the direction from the boss to the kid and sets the apples direction to that, and then sets this alarm to go off again 1/2 of a second later. This has the effect of shooting 2 apples at the kid every second. We have to re-set the alarm at the end to make sure it goes off again, otherwise you'll only get one attack!
Go ahead and try out your boss now - and don't get Rekt by the apples!
Apple-splosionsApple explosions (or apple-splosions (c) (tm)) are when you see a giant ring of apples fly out of miku's mouth, hand, or other uh... body parts. To make this happen, start off by making an Alarm 1 event, and in the creation event, change
alarm[0] to
alarm[1] so that Alarm 1 goes off instead of Alarm 0. Leave Alarm 0's actions alone for now, we'll revisit it later.
In Alarm 1, give it the following code:
appleCount=8;
dir=random(360);
for (i=0;i<appleCount;i+=1) {
apple=instance_create(x,y,objApple);
apple.speed=6;
apple.direction=dir;
dir += 360/appleCount;
}
alarm[1]=room_speed;
Let's do another line by line analysis. The first 2 lines declare two variables, appleCount & dir.
appleCount will represent how many apples you want to shoot; we choose 8 for now.
dir will represent the direction of the current apple, in degrees.
random(x) returns a random value between 0 and x, so we start off with a random angle for the apples.
Then, we start a
for loop, which is a common construct in programming languages. This for statement starts with a variable named "i" with a value of zero, will loop as long as "i" is less than
appleCount, and will add one to "i" every pass through the loop. The code we want to execute in the for loop is in the curly brackets {} which we'll call the
body of the for loop, let's investigate that next.
The body of the loop should look really familiar; it's basically the same as the targetted apples, with one exception. Here, the direction is not towards the player, but rather is calculated differently. We start with
dir equal to that random value, and increase it by
360 divided by appleCount every iteration of the loop. This ensures that we get apples equally spaced along a circle. For instance, if dir were zero to start with, we would get 8 apples with directions 0, 45, 90, 135, 180, 225, 270, and 315 degrees.
Finally, we set the alarm to go off again in one second, like in the previous example.
Well, that was a mouthful. Go ahead and try out your boss now, every second he'll blast a ring of apples at you in a random orientation!
Check back later, and I'll go over more interesting patterns if I ever get a break from making my own fangames


Thanks Wolfy